在软件工程的动态世界中,跟上编程语言的最新进展对于制定强大而高效的解决方案至关重要。随着 C++ 标准的每次新迭代,都会引入大量现代功能,彻底改变我们编写代码的方式。在本文中,我们将揭秘最新标准(C++11 及更高版本)中引入的一些最重要的现代 C++ 功能。从智能指针到 lambda,将语义转移到 constexpr 函数,我们将通过实际示例和实际用例探索这些功能,使软件工程师能够在其项目中充分利用现代 C++ 的潜力。
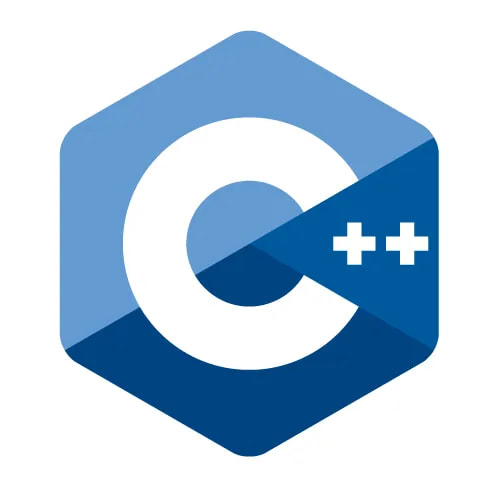
智能指针
智能指针是原始指针的智能包装器,用于管理动态分配对象的内存。它们提供自动内存管理,降低内存泄漏的风险并提高代码安全性。示例包括 std::unique_ptr、std::shared_ptr 和 std::weak_ptr。让我们看看 std::unique_ptr 在实践中是如何工作的:
#include <memory> #include <iostream>int main() { // Creating a unique pointer to manage dynamically allocated memory std::unique_ptr<int> ptr = std::make_unique<int>(42); // Accessing the value std::cout << “Value managed by unique_ptr: ” << *ptr << std::endl; return 0; } |
拉姆达
Lambda 是匿名函数,可以从其周围范围捕获变量。它们提供了一种简洁而灵活的方式来定义内联函数,特别是与算法和回调函数一起使用。让我们看一个将 lambda 函数与 std::sort 结合使用的简单示例:
#include <algorithm> #include <vector> #include <iostream>int main() { std::vector<int> numbers = {3, 1, 4, 1, 5, 9, 2, 6, 5};// Sorting the vector using a lambda function std::sort(numbers.begin(), numbers.end(), [](int a, int b) { return a < b; }); // Printing the sorted vector for (const auto& num : numbers) { std::cout << num << ” “; } std::cout << std::endl; return 0; } |
移动语义
移动语义允许将资源(例如内存)从一个对象有效转移到另一个对象,从而避免不必要的复制并提高性能。它们对于优化资源密集型操作的性能特别有用。让我们看看移动语义如何与 std::move 一起使用:
#include <iostream> #include <vector>int main() { std::vector<int> source = {1, 2, 3, 4, 5}; // Moving the content of source to destination std::vector<int> destination = std::move(source); // Printing the content of destination for (const auto& num : destination) { std::cout << num << ” “; } std::cout << std::endl; // Source vector is now empty std::cout << “Size of source vector: ” << source.size() << std::endl; return 0; } |
常量表达式函数
Constexpr 函数在编译时求值,允许计算编译时已知的值。它们可以创建更高效、更灵活的代码,尤其是在性能关键的场景中。以下是用于计算数字阶乘的 constexpr 函数的示例:
#include <iostream> constexpr int factorial(int n) { |
最新标准中引入的现代 C++ 功能改变了我们编写代码的方式,提供了更高的安全性、性能和灵活性。通过理解和利用智能指针、lambda、移动语义和constexpr函数等功能,软件工程师可以编写更高效和可维护的代码,从而提高生产力和更高质量的软件解决方案。在您的项目中采用这些现代 C++ 功能,以释放其全部潜力,并在不断发展的软件开发领域保持领先地位。